PCjr programming tests
Moderator: Ice Cream Jonsey
- Ice Cream Jonsey
- Posts: 30065
- Joined: Sat Apr 27, 2002 2:44 pm
- Location: Colorado
- Contact:
PCjr programming tests
I am trying to see if I can use chatGPT to help me write code that works on a PCjr.
I asked it to make something that showed all 16 colors the PCjr can do at once, at its smallest resolution. But before we go there, I tried to compile this with MASM 4.
That really didn't work, so I found a copy of MASM 5 and that seemed to at least compile what chatGPT was giving me. Unfortunately, when I asked it to give me a program that turned the screen blue and waited for the spacebar to exit, it didn't work. I am not 100% sure it knows about PCjr color commands.
I asked it to make something that showed all 16 colors the PCjr can do at once, at its smallest resolution. But before we go there, I tried to compile this with MASM 4.
That really didn't work, so I found a copy of MASM 5 and that seemed to at least compile what chatGPT was giving me. Unfortunately, when I asked it to give me a program that turned the screen blue and waited for the spacebar to exit, it didn't work. I am not 100% sure it knows about PCjr color commands.
the dark and gritty...Ice Cream Jonsey!
- Ice Cream Jonsey
- Posts: 30065
- Joined: Sat Apr 27, 2002 2:44 pm
- Location: Colorado
- Contact:
Re: PCjr programming tests
Here is the code that I am running at the moment. I reached out on the Brutman PCjr site for help.
Code: Select all
.model small
.data
.code
.stack 100h ; Define a stack segment with 256 bytes (100h in hexadecimal)
org 100h
start:
; Your code here
wait_for_space:
mov ah, 0
int 16h ; Wait for a key press
cmp al, 32 ; Check if the key pressed is the space bar (ASCII code 32)
jne wait_for_space ; If not, keep waiting
mov ax, 3 ; Set video mode 3 (80x25 text mode)
int 10h
mov ax, 4C00h ; DOS function: Terminate program
int 21h
end start
the dark and gritty...Ice Cream Jonsey!
- Ice Cream Jonsey
- Posts: 30065
- Joined: Sat Apr 27, 2002 2:44 pm
- Location: Colorado
- Contact:
Re: PCjr programming tests
I got some help at a PCjr forum, and the person was wonderful and explained what chatGPT did wrong. I am amazed chatGPT got as close as it did. I am going to write this process up, but here's a picture of a PCjr showing a painted blue screen at its native 160x200x16 color resolution.
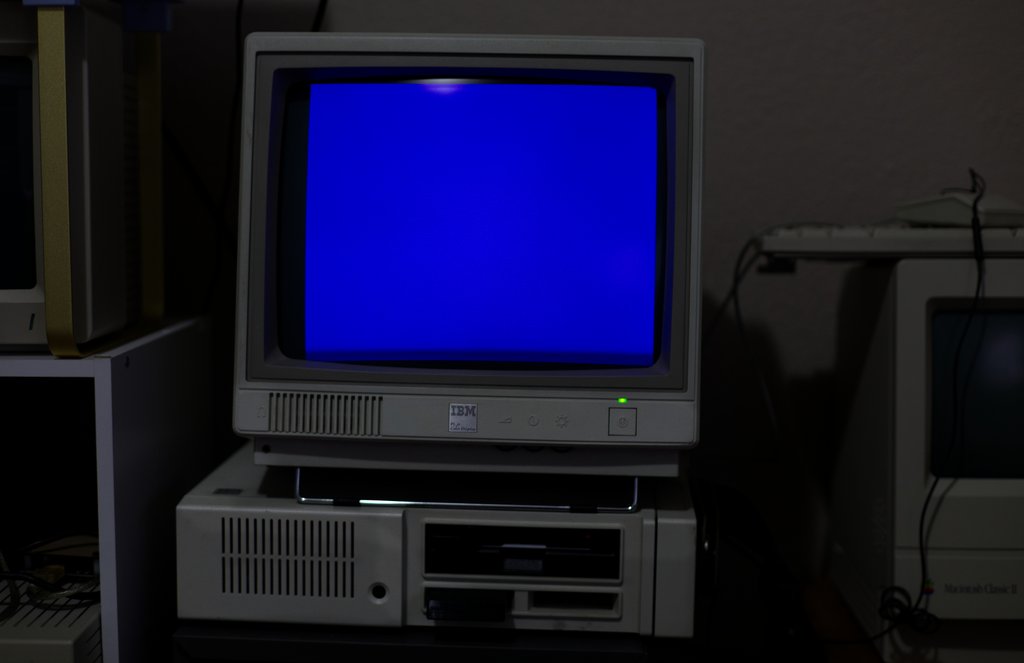
I am going to try to see if I can do some other things. It would be nice if I could get it to cycle all the colors and then also display them all at the screen at once.
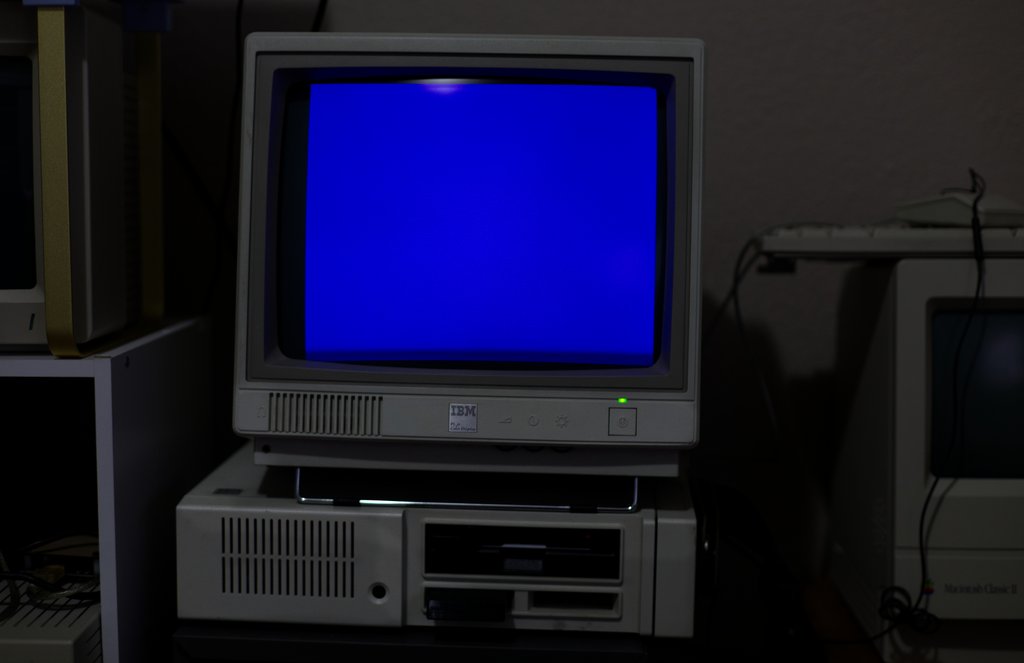
I am going to try to see if I can do some other things. It would be nice if I could get it to cycle all the colors and then also display them all at the screen at once.
the dark and gritty...Ice Cream Jonsey!
- Ice Cream Jonsey
- Posts: 30065
- Joined: Sat Apr 27, 2002 2:44 pm
- Location: Colorado
- Contact:
Re: PCjr programming tests
I wrote some code to wait for the enter key, and then paint the screen cyan. But I tried to use a global variable to store the color information and that is not working. I am not sure why. I am declaring it in the .data section as such:
Which is 00010001, or 17 in decimal (17d). I tried to store it as hexidecimal as well. When it attempts to put that value into the al register and paint the screen, I see a magenta background with the > character in cyan repeated. So something is getting corrupted somewhere. I'll post my current code afterwards.
Code: Select all
color_index db 17d
the dark and gritty...Ice Cream Jonsey!
- Ice Cream Jonsey
- Posts: 30065
- Joined: Sat Apr 27, 2002 2:44 pm
- Location: Colorado
- Contact:
Re: PCjr programming tests
Code: Select all
.model small
.data
color_index db 17 ; Start with blue.
.code
.stack 100h ; Define a stack segment with 256 bytes (100h in hexadecimal)
org 100h
start:
mov ax, 0010h ; Set video mode 10h (160x200, 16 colors)
int 10h ; This is the software interrupt that triggers a BIOS video service call.
color_loop:
; Set the entire screen to blue (color index 1)
mov ax, 0B800h ; Segment address for PCjr video memory
mov es, ax ; Sets the Extra Segment register.
xor di, di ; Destination offset (start of video memory)
mov cx, 04000h ; Number of bytes (160x200 pixels)
mov al,17 ; Blue (decimal 17, hex 11h, binary 0001 0001).
;mov al,22h ; Green? (decimal 34, hex 22h, binary 0010 0010).
cld ; Clear direction flag for forward string operation
; repeat storebyte. Repeats it the number of times specified by the cx register.
rep stosb ; Fill screen with blue.
; Wait for Enter key (carriage return)
wait_for_enter:
mov ah, 0
int 16h ; Wait for a key press
cmp al, 13 ; Check if the key pressed is the Enter key (ASCII code 13)
jne wait_for_enter ; If not, keep waiting
; BLUE 17, GREEN 34, CYAN 51, next color is 68
; Set the entire screen to blue (color index 1)
mov ax, 0B800h ; Segment address for PCjr video memory
mov es, ax ; Sets the Extra Segment register.
xor di, di ; Destination offset (start of video memory)
mov cx, 04000h ; Number of bytes (160x200 pixels)
mov al,51 ; Blue (decimal 17, hex 11h, binary 0001 0001).
cld ; Clear direction flag for forward string operation
; repeat storebyte. Repeats it the number of times specified by the cx register.
rep stosb ; Fill screen with blue.
; Wait for Enter key (carriage return)
wait_for_enter2:
mov ah, 0
int 16h ; Wait for a key press
cmp al, 13 ; Check if the key pressed is the Enter key (ASCII code 13)
jne wait_for_enter2 ; If not, keep waiting
; Cycle to the next color
; mov al, [color_index] ; Load the current color index
; add al, 17 ; Increment by 17 in decimal for the next color
; cmp al, 68 ; Check if we've reached the end of the colors
; jae - jump above or equal
; jae wait_for_space ; If so, jump to wait_for_space
; mov [color_index], al ; Store the updated color index
; jmp color_loop ; Repeat the color cycle loop
wait_for_space:
mov ah, 0
int 16h ; Wait for a key press
cmp al, 32 ; Check if the key pressed is the space bar (ASCII code 32)
jne wait_for_space ; If not, keep waiting
mov ax, 3 ; Set video mode 3 (80x25 text mode)
int 10h
mov ax, 4C00h ; DOS function: Terminate program
int 21h
end start
the dark and gritty...Ice Cream Jonsey!
- Ice Cream Jonsey
- Posts: 30065
- Joined: Sat Apr 27, 2002 2:44 pm
- Location: Colorado
- Contact:
Re: PCjr programming tests
Things I would like to do:
1. Instead of painting the entire screen, divide it into 16 colors and show them all at the same time
2. Use functions for painting the screen, taking in arguments for what pixels to paint and what color
3. Get myself good code for the global variable. Why is it messed up?
I would love to make a game loop where I just have a little space ship shooting aliens.
1. Instead of painting the entire screen, divide it into 16 colors and show them all at the same time
2. Use functions for painting the screen, taking in arguments for what pixels to paint and what color
3. Get myself good code for the global variable. Why is it messed up?
I would love to make a game loop where I just have a little space ship shooting aliens.
the dark and gritty...Ice Cream Jonsey!
- RealNC
- Posts: 2289
- Joined: Wed Mar 07, 2012 4:32 am
Re: PCjr programming tests
At this point, you should probably download Open Watcom and make your life easier :P
- Jizaboz
- Posts: 5420
- Joined: Tue Jan 31, 2012 2:00 pm
- Location: USA
- Contact:
Re: PCjr programming tests
I'm at the exact same stage (and have been for years) with Vectrex programming via the VecFever. "Ok I got screens, I got animations, now I want to have a simple game loop for a dude shooting objects flying at him."
(╯°□°)╯︵ ┻━┻
- Ice Cream Jonsey
- Posts: 30065
- Joined: Sat Apr 27, 2002 2:44 pm
- Location: Colorado
- Contact:
Re: PCjr programming tests
I haven't had a chance to come back. I kind of want to see if chatGPT4 is any smarter.
the dark and gritty...Ice Cream Jonsey!
- Ice Cream Jonsey
- Posts: 30065
- Joined: Sat Apr 27, 2002 2:44 pm
- Location: Colorado
- Contact:
Re: PCjr programming tests
This would be coding g in C, right?RealNC wrote: Wed Sep 20, 2023 6:55 am At this point, you should probably download Open Watcom and make your life easier :P
the dark and gritty...Ice Cream Jonsey!
- RealNC
- Posts: 2289
- Joined: Wed Mar 07, 2012 4:32 am
Re: PCjr programming tests
Yeah. Or C++.Ice Cream Jonsey wrote: Thu Sep 28, 2023 10:17 pmThis would be coding g in C, right?RealNC wrote: Wed Sep 20, 2023 6:55 am At this point, you should probably download Open Watcom and make your life easier :P
- Ice Cream Jonsey
- Posts: 30065
- Joined: Sat Apr 27, 2002 2:44 pm
- Location: Colorado
- Contact:
Re: PCjr programming tests
I think if I am actually gonna do anything with this, I'll have to go that route. There is some sample code in the book "Machine Language" ... ok, lemme break out of quotes because I don't remember the name of the book. There is some sample Assembly in a Machine Language book for the IBM PC and PCjr that might help me do some movement.RealNC wrote: Thu Sep 28, 2023 11:24 pmYeah. Or C++.Ice Cream Jonsey wrote: Thu Sep 28, 2023 10:17 pmThis would be coding g in C, right?RealNC wrote: Wed Sep 20, 2023 6:55 am At this point, you should probably download Open Watcom and make your life easier :P
But I thank PICO8 for having it "click" how motion works in games for me. Can we all thank PICO8?
the dark and gritty...Ice Cream Jonsey!
- Ice Cream Jonsey
- Posts: 30065
- Joined: Sat Apr 27, 2002 2:44 pm
- Location: Colorado
- Contact:
Re: PCjr programming tests
Let's figure out why this doesn't work.

"GIFV." Ok. I am sure that will work, imgur.
"GIFV." Ok. I am sure that will work, imgur.
the dark and gritty...Ice Cream Jonsey!
- Ice Cream Jonsey
- Posts: 30065
- Joined: Sat Apr 27, 2002 2:44 pm
- Location: Colorado
- Contact:
Re: PCjr programming tests
Thanks, imgur. What a piece of shit site.
the dark and gritty...Ice Cream Jonsey!
- Ice Cream Jonsey
- Posts: 30065
- Joined: Sat Apr 27, 2002 2:44 pm
- Location: Colorado
- Contact: